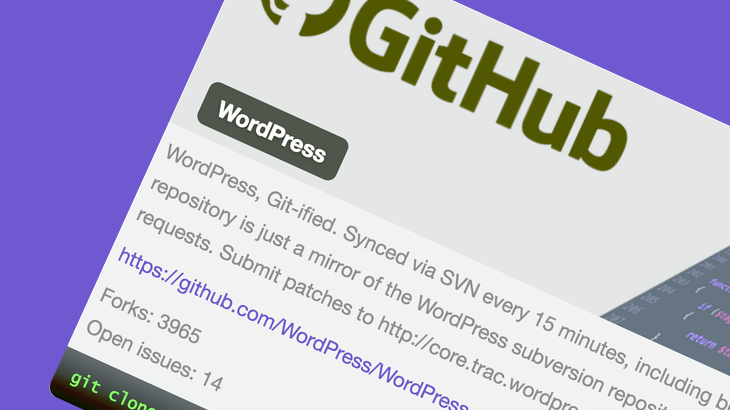
WordPress
GitHub Repository Shortcode
GitHub
[gitrepo author=”f13dev” repo=”wp-github-repo-shortcode”]
Introduction
Are you a programmer who uses GitHub? Do you want to add information about a repository to your WordPress powered blog?
If you answered yes to the above then ‘GitHub Repo Shortcode’ may be for you. This plugin utilises the GitHub API to retrieve information about a repository and display information about the repo on your website.
Features
Easily display a showcase of your GitHub repository on your WordPress powered website. Each showcase includes the following features:
- Cached using Transient, the cache timout can be adjusted on the admin page
- A styled widget with a GitHub banner
- Repository description
- Link to the repository on GitHub
- Statistics for: Forks, Stars & Open issues
- A link to the latest tag (if one exists)
- Shows the code to clone the repository (using git clone …)
- Can be used with or without a GitHub API Token
Although this plugin will work without a GitHub API Token the number of API calls are restricted and as such may cause information not to appear if multiple instances of the shortcode are used, or if the cache timeout is set to a low time. It is therefor recommended that an API Token is used.
Installation
- Upload the plugin files to the `/wp-content/plugins/plugin-name` directory, or install the plugin through the WordPress plugins screen directly.
- Activate the plugin through the ‘Plugins’ screen in WordPress
- Add the shortcode [[gitrepo author=”f13dev” repo=”wp-github-repo-shortcode”]] to the desired location
- If desired, add a GitHub API Token and/or alter the cache timeout on the admin page Settings->F13 GitHub Repo Shotcode
Usage
Once ‘GitHub Repo Shortcode’ has been installed on your WordPress powered website and the plugin has been activated:
- Find the author name and repo name of the repository that you wish to use the shortcode with. This can be found from the URL of the GitHub repository; e.g. https://github.com/f13dev/wp-github-repo-shortcode has an author of ‘f13dev and a repo of ‘wp-github-repo-shortcode.
- On the page or blog post where you wish to showcase your GitHub repository add the following shortcode: [[gitrepo author=”f13dev” repo=”wp-github-repo-shortcode”]]
- Save the page or blog post, your GitHub repository showcase should now be displayed on your page or blog post.
Add a GitHub API Token (Optional):
- Visit: https://github.com/settings/tokens
- Click the ‘Generate New Token’ button in the top right corner of the page
- Enter your GitHub password for security reasons
- Enter a token description. For example: API access from my blog
- Click the ‘Generate Token’ button at the bottom of the page
- Copy and paste the newly created token to the settings page in the WordPress admin area under Settings->F13 GitHub Repo Shortcode
- Click save changes
Code
Plugin code:
<?php /* Plugin Name: GitHub Repository Shortcode Plugin URI: http://f13dev.com Description: This plugin enables you to enter shortcode on any page or post in your blog to show information and statistics about a repository on GitHub. Version: 1.0 Author: Jim Valentine - f13dev Author URI: http://f13dev.com Text Domain: f13-github-repo-shortcode License: GPLv3 */ /* Copyright 2016 James Valentine - f13dev (jv@f13dev.com) This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 3 of the License, or any later version. This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA */ // Register the shortcode add_shortcode( 'gitrepo', 'f13_github_repo_shortcode'); // Register the css add_action( 'wp_enqueue_scripts', 'f13_github_repo_style'); // Register the admin page add_action('admin_menu', 'f13_grs_create_menu'); /** * The main function to create the shortcode results * storing or retreiving the data from cache * @param [type] $atts [description] * @param [type] $content [description] * @return [type] [description] */ function f13_github_repo_shortcode( $atts, $content = null ) { // Get the attributes extract( shortcode_atts ( array ( 'author' => 'none', 'repo' => 'none' // Default slug won't show a plugin ), $atts )); // Set the cache name for this instance of the shortcode $cache = get_transient('wpgrs' . md5(serialize($atts))); if ($cache) { // If the cache exists, return it rather than re-creating it return $cache; } else if ($author != null || $repo != null) { // Get the plugin settings variables for timeout and token $timeout = esc_attr( get_option('cache_timeout')) * 60; // If the timeout is set to 0, or is not a number, change it to 1 second, so effectively not // caching, but saves a cache that doesnt timeout if the setting is changed later if ($timeout == 0 || !is_numeric($timeout)) { $timeout = 1; } $token = esc_attr( get_option('token')); // If the cache doesn't exist, create it and return the shortcode // Generate the API results for the repository $repository = f13_get_github_api('https://api.github.com/repos/' . $author . '/' . $repo, $token); // Generate the API results for the tags $tags = f13_get_github_api('https://api.github.com/repos/' . $author . '/' . $repo . '/tags', $token); // Get the response of creating the shortcode $response = f13_format_github_repo($repository, $tags); // Store the output of the shortcode into the cache set_transient('wpgrs' . md5(serialize($atts)), $response, $timeout); // Return the response return $response; } else { return 'The author and repo attributes are required, enter [[gitrepo author="anAuhor" repo="aRepo"]] to use this shortcode.'; } } /** * A function to register the stylesheet * @return [type] [description] */ function f13_github_repo_style() { wp_register_style( 'f13github-style', plugins_url('wp-github-repo-shortcode.css', __FILE__) ); wp_enqueue_style( 'f13github-style' ); } /** * A function to retrieve the repository information via * the GitHub API. * @param $author The author of the GitHub repository * @param $repo The name of the GitHub repository * @param $token The API token used to access the GitHub API * @return A decoded array of information about the GitHub repository */ function f13_get_github_api($url, $token) { // Start curl $curl = curl_init(); // Set curl options curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HTTPGET, true); // Check if a token is set if (preg_replace('/\s+/', '', $token) != '' || $token != null) { // If a token is set attempt to send it in the header curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Accept: application/json', 'Authorization: token ' . $token )); } else { // If no token is set, send the header as unauthenticated, // some features may not work and a lower rate limit applies. curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Accept: application/json' )); } // Set the user agent curl_setopt($curl, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.13) Gecko/20080311 Firefox/2.0.0.13'); // Set curl to return the response, rather than print it curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); // Get the results $result = curl_exec($curl); // Close the curl session curl_close($curl); // Decode the results $result = json_decode($result, true); // Return the results return $result; } /** * A function to convert an array of informatino regarding a GitHub * repository and return a HTML & CSS formatted widget. * @param [type] $results An array of information regarding a GitHub repository * @return [type] A HTML formatted string of information regarding a GitHub repository */ function f13_format_github_repo($repository, $tags) { $latestTag = f13_get_github_latest_tag($tags); if ($latestTag != 'None') { $latestTag = '<a href="https://github.com/' . $repository['full_name'] . '/releases/tag/' . $latestTag . '">' . $latestTag . '</a>'; } $string = ' <div class="gitContainer"> <div class="gitHeader"> <span class="gitTitle"> <a href="' . $repository['html_url'] . '">'. $repository['name'] . '</a> </span> </div>'; if ($repository['description'] != null) { $string .= ' <div class="gitDescription"> ' . $repository['description'] . ' </div>'; } $string .=' <div class="gitLink"> <a href="' . $repository['html_url'] . '">' . $repository['html_url'] . '</a> </div> <div class="gitStats"> <div class="gitForks"> Forks: ' . $repository['forks_count'] . ' </div> <div class="gitStars"> Stars: ' . $repository['stargazers_count'] . ' </div> <div class="gitOpenIssues"> Open issues: ' . $repository['open_issues_count'] . ' </div> <div class="gitLatestTag"> Latest tag: ' . $latestTag . ' </div> </div> <div class="gitClone"> git clone ' . $repository['clone_url'] . ' </div> </div> '; return $string; } /** * A function to return the latest tag name * if one or more tags exist, otherwise returns * none. * @param [type] $tags [description] * @return [type] [description] */ function f13_get_github_latest_tag($tags) { if (count($tags) > 0) { return $tags[0]['name']; } else { return 'None'; } } /** * Functions to create the backend */ /** * A function to register the menu page * @return [type] [description] */ function f13_grs_create_menu() { // Create the top-level menu add_options_page('F13Devs GitHub Repo Shortcode Settings', 'F13 GitHub Repo Shortcode', 'administrator', 'f13-github-repo-shortcode', 'f13_grs_settings_page'); // Retister the Settings add_action( 'admin_init', 'f13_grs_settings'); } /** * A function to register the settings group * @return [type] [description] */ function f13_grs_settings() { // Register settings for token and timeout register_setting( 'f13-grs-settings-group', 'token'); register_setting( 'f13-grs-settings-group', 'cache_timeout'); } /** * A function to create the settings page * @return [type] [description] */ function f13_grs_settings_page() { ?> <div class="wrap"> <h2>GitHub Settings</h2> <p> Welcome to the settings page for GitHub Repo Shortcode. </p> <p> This plugin can be used without an API token, although it is recommended to use one as the number of API calls is quite restrictive without one. </p> <p> To obtain a GitHub API token: <ol> <li> Log-in to your GitHub account. </li> <li> Visit <a href="https://github.com/settings/tokens">https://github.com/settings/tokens</a>. </li> <li> Click the 'Generate new token' button at the top of the page/ </li> <li> Re-enter your GitHub password for security. </li> <li> Enter a description, such as 'my wordpress site'. </li> <li> Click the 'Generate token' button at the bottom of the page, no other setting changes are required. </li> <li> Copy and paste your new API token. Please note, your access token will only be visible once. </li> </ol> </p> <form method="post" action="options.php"> <?php settings_fields( 'f13-grs-settings-group' ); ?> <?php do_settings_sections( 'f13-grs-settings-group' ); ?> <table class="form-table"> <tr valign="top"> <th scope="row"> GitHub Token </th> <td> <input type="password" name="token" value="<?php echo esc_attr( get_option( 'token' ) ); ?>" style="width: 50%;"/> </td> </tr> <tr valign="top"> <th scope="row"> Cache timeout (minutes) </th> <td> <input type="number" name="cache_timeout" value="<?php echo esc_attr( get_option( 'cache_timeout' ) ); ?>" style="width: 75px;"/> </td> </tr> </table> <?php submit_button(); ?> </form> <h3>Shortcode example</h3> <p> If you wish to display a widget showing details of a project at: https://github.com/f13dev/wp-github-repo-shortcode use the following shortcode: </p> <p> [[gitrepo author="f13dev" repo="wp-github-repo-shortcode"]] </p> </div> <?php }
CSS:
.gitContainer { border-radius: 10px; background: #fff; /*border: 1px solid grey;*/ background: #eee; } .gitIcon { background-image: url('img/git_logo.png'); width: 32px; height: 32px; position: absolute; margin-left: 0.15em; margin-top: 0.15em; } .gitHeader { width: 100%; height: 200px; background: url('img/banner.png'); border-radius: 10px 10px 0 0; background-repeat: none; } .gitTitle { font-size: 20px; line-height: 1em; padding: 8px 15px; background: rgba( 30, 30, 30, 0.9); text-shadow: 0 1px 3px rgba( 0, 0, 0, 0.4); border-radius: 8px; font-family: "Helvetica Neue", sans-serif; font-weight: bold; margin-top: 200px; margin-left: 20px; position: relative; top: 160px; } .gitTitle a { text-decoration: none; color: #fff; } .gitTitle a:hover { color: #fff; text-decoration: underline; } .gitDescription { padding: 0.5em 0.5em 0 0.5em; font-size: 1.2em; } .gitLink { padding: 0.5em 0.5em 0 0.5em; font-size: 1.2em; } .gitStats { padding: 0.5em; font-size: 1.2em; } .gitStats > div { width: 40%; min-width: 220px; display: inline-block; } .gitClone { /* Permalink - use to edit and share this gradient: http://colorzilla.com/gradient-editor/#45484d+0,000000+100;Black+3D+%231 */ background: rgb(69,72,77); /* Old browsers */ background: -moz-linear-gradient(top, rgba(69,72,77,1) 0%, rgba(0,0,0,1) 100%); /* FF3.6-15 */ background: -webkit-linear-gradient(top, rgba(69,72,77,1) 0%,rgba(0,0,0,1) 100%); /* Chrome10-25,Safari5.1-6 */ background: linear-gradient(to bottom, rgba(69,72,77,1) 0%,rgba(0,0,0,1) 100%); /* W3C, IE10+, FF16+, Chrome26+, Opera12+, Safari7+ */ filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#45484d', endColorstr='#000000',GradientType=0 ); /* IE6-9 */ color: #33cc33; padding: 10px 15px; border-radius: 0 0 10px 10px; font-family: Lucida Console,Lucida Sans Typewriter,monaco,Bitstream Vera Sans Mono,monospace; } .gitURL a:hover { text-decoration: underline; }
1 comments on "WordPress Plugin: GitHub Repo Shotcode"