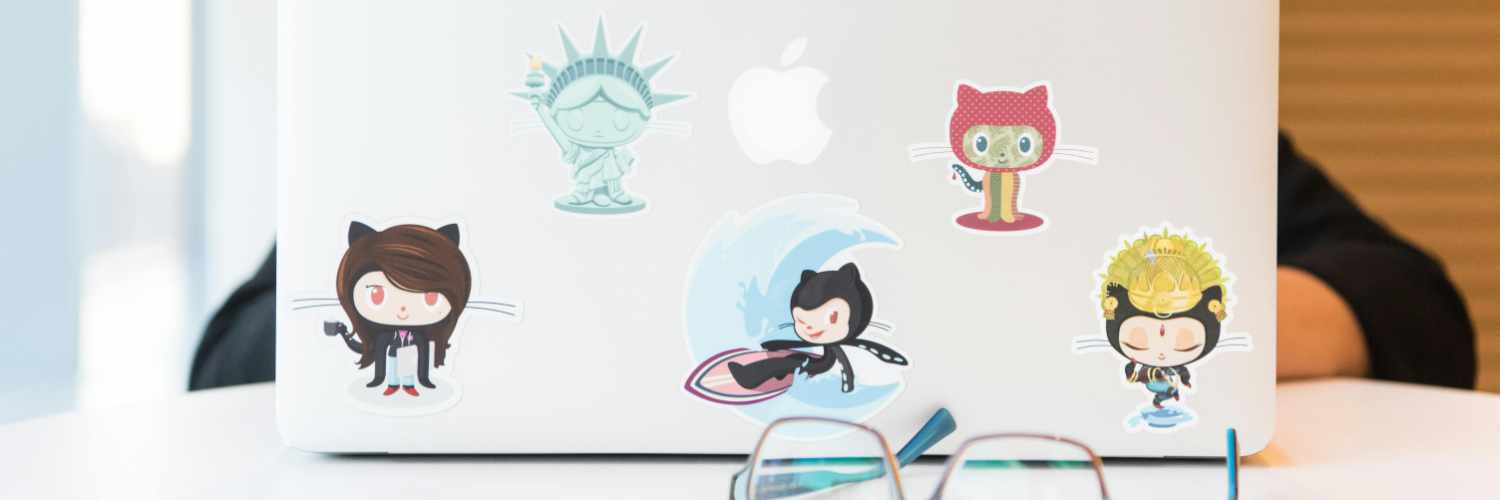
Gist shortcode examples
Working examples
Result of: [gist id="fc53666cfbde382ca6a5ae1c519dc65a"]
Gist created by: f13dev
Created: July 06, 2016 - 10:05am
Last edited: July 06, 2016 - 10:08am
Last edited: July 06, 2016 - 10:08am
Description: N/A
Files (2)
wp-api.class.php (6.66kb) Download file<?phpwp-api.test.php (1.16kb) Download file
class wordpress_pluing_information
{
// Variable to store plugin name
var $slug;
var $results;
function wordpress_pluing_information($aSlug)
{
// Set the pluignName of the new object to the argument
$this->slug = $aSlug;
// Generate results
$this->getResults();
}
private function getResults()
{
// start curl
$curl = curl_init();
// set the curl URL
$url = 'https://api.wordpress.org/plugins/info/1.0/' . $this->slug . '.json';
// Set curl options
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_HTTPGET, true);
// Set the user agent
curl_setopt($curl, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.13) Gecko/20080311 Firefox/2.0.0.13');
// Set curl to return the response, rather than print it
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
// Get the results and store the XML to results
$this->results = json_decode(curl_exec($curl), true);
// Close the curl session
curl_close($curl);
}
function getName()
{
return $this->results['name'];
}
function getSlug()
{
return $this->results['slug'];
}
function getPluginURL()
{
return 'https://wordpress.org/plugins/' . $this->getSlug() . '/';
}
function getVersion()
{
return $this->results['version'];
}
function getAuthor()
{
return $this->results['author'];
}
function getAuthorURL()
{
return $this->results['author_profile'];
}
function getAuthorLink()
{
return $this->results['contributors'][0];
}
function getContributorsList()
{
$list = '<ul>';
foreach ($this->results['contributors'] as $key => $value)
{
$list .= '<li><a href="' . $value . '">' . $key . '</a></li>';
}
$list .= '</ul>';
return $list;
}
function getVersionRequired()
{
return $this->results['requires'];
}
function getVersionTestedOn()
{
return $this->results['tested'];
}
function getRating()
{
return $this->results['rating'] / 20;
}
function getRatingNumber()
{
return $this->results['num_ratings'];
}
function getRatingStars()
{
for ($x = 1; $x < $this->getRating(); $x++ )
{
echo '<img src="img/star-full.png" />';
}
if (strpos($this->getRating(), '.'))
{
echo '<img src="img/star-half.png" />';
$x++;
}
while ($x <= 5)
{
echo '<img src="img/star-empty.png" />';
$x++;
}
}
function getNumberDownloads()
{
return $this->results['downloaded'];
}
function getLastUpdate()
{
$date = explode ('-', explode(' ', $this->results['last_updated'])[0]);
$string = $date[2] . ' ' . $this->convertNumberToMonth($date[1]) . ' ' . $date[0];
return $string;
}
function getCreationDate()
{
$date = explode ('-', explode(' ', $this->results['added'])[0]);
$string = $date[2] . ' ' . $this->convertNumberToMonth($date[1]) . ' ' . $date[0];
return $string;
}
function getBannerURL()
{
$baseURL = 'https://ps.w.org/' . $this->getSlug() . '/assets/banner-772x250';
if ($this->remoteFileExists($baseURL . '.jpg'))
{
return $baseURL . '.jpg';
}
else if ($this->remoteFileExists($baseURL . '.png'))
{
return $baseURL . '.png';
}
else
{
return 'img/default_banner.png';
}
}
function getBannerImage()
{
return '<img src="' . $this->getBannerURL() . '" />';
}
function getIconURL()
{
$baseURL = 'https://ps.w.org/' . $this->getSlug() . '/assets/icon-128x128';
if ($this->remoteFileExists($baseURL . '.jpg'))
{
return $baseURL . '.jpg';
}
else if ($this->remoteFileExists(baseURL . '.png'))
{
return $baseURL . '.png';
}
else
{
return 'img/default_icon.png';
}
}
function getIconImage()
{
return '<img src="' . $this->getIconURL() . '" />';
}
function getShortDescription()
{
return $this->results['short_description'];
}
function getDownloadURL()
{
return $this->results['download_link'];
}
function getDownloadLink()
{
return '<a href="' . $this->getDownloadURL() . '">Download</a>';
}
function getTagsList()
{
$string = '<ul>';
foreach ($this->results['tags'] as $key => $value)
{
$string .= '<li>' . $value . '</li>';
}
$string .= '</ul>';
return $string;
}
function getDonateURL()
{
return $this->results['donate_link'];
}
function getDonateLink()
{
return '<a href="' . $this->getDonateURL() . '">Donate</a>';
}
private function convertNumberToMonth($month)
{
if ($month == 01)
{
return "January";
}
else if ($month == 02)
{
return "February";
}
else if ($month == 03)
{
return "March";
}
else if ($month == 04)
{
return "April";
}
else if ($month == 05)
{
return "May";
}
else if ($month == 06)
{
return "June";
}
else if ($month == 07)
{
return "July";
}
else if ($month == 08)
{
return "August";
}
else if ($month == 09)
{
return "September";
}
else if ($month == 10)
{
return "October";
}
else if ($month == 11)
{
return "November";
}
else if ($month == 12)
{
return "December";
}
else
{
return null;
}
}
private function remoteFileExists($url)
{
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_NOBODY, true);
$result = curl_exec($curl);
if ($result != false)
{
if (curl_getinfo($curl, CURLINFO_HTTP_CODE) == 200)
{
return true;
}
}
else
{
return false;
}
}
}
<?php
// Require the api PHP file
require_once('wp-api.class.php');
// Create a new object for the API
$wpapi = new wordpress_pluing_information('WP-Twitter-profile-widget');
// Returning results
echo 'Name: ' . $wpapi->getName() . '<br/>';
echo 'Slug: ' . $wpapi->getSlug() . '<br/>';
echo 'Version: ' . $wpapi->getVersion() . '<br/>';
echo 'Author' . $wpapi->getAuthor() . '<br/>';
echo 'Author profile: ' . $wpapi->getAuthorLink() . '<br/>';
echo 'Contributors: ' . $wpapi->getContributorsList();
echo 'Requires version: ' . $wpapi->getVersionRequired() . '<br/>';
echo 'Tested on: ' . $wpapi->getVersionTestedOn . '<br/>';
echo 'Rating: ' . $wpapi->getRatingStars() . '<br/>';
echo 'Downloaded: ' . $wpapi->getNumberDownloads() . '<br/>';
echo 'Last updated: ' . $wpapi->getLastUpdate() . '<br/>';
echo 'Added: ' . $wpapi->getCreationDate() . '<br/>';
echo 'Header image: ' . $wpapi->getBannerImage() . '<br/>';
echo 'Icon: ' . $wpapi->getIconImage() . '<br/>';
echo 'Short description: ' . $wpapi->getShortDescription() . '<br/>';
echo 'Download: ' . $wpapi->getDownloadLink() . '<br/>';
echo 'Tags: ' . $wpapi->getTagsList();
echo 'Donate link: ' . $wpapi->getDonateLink() . '<br/>';
Comments: 0
Result of: [gist id="85ea6e90e79cad8dfe7c7cd699b13f41"]
Gist created by: f13dev
Created: June 27, 2016 - 07:15pm
Last edited: June 29, 2016 - 10:37am
Last edited: June 29, 2016 - 10:37am
Description: Converts a plain text Twitter tweet into html to <a> tags for URLs, #links and @links
Files (1)
getLinksFromTwitterText.php (0.81kb) Download file<?php
/**
* Takes a string argument, which may include URLs, twitter #links or twitter @links,
* imbeds html <a> tags into the string and returns it.
*
* @param [type] $string [description]
* @return [type] $string [description]
*/
function getLinksFromTwitterText($string)
{
// Converts a plain text url to a hyperlink
$string = preg_replace('~(?:(https?)://([^\s<]+)|(www\.[^\s<]+?\.[^\s<]+))(?<![\.,:])~i', '<a href="$0" ' . $target . ' " title="$0">$0</a>', $string);
// Converts hashtags to a link
$string = preg_replace("/#([A-Za-z0-9\/\.]*)/", "<a href=\"http://twitter.com/search?q=$1\" " . $target . " >#$1</a>", $string);
// Converts @user to a link
$string = preg_replace("/@([A-Za-z0-9\/\.]*)/", "<a href=\"http://www.twitter.com/$1\" $target >@$1</a>", $string);
return $string;
}
Comments: 0
Broken example
Result of: [gist id="not_found"]
Not Found
Attributes
user is the github user, repo is the users repo name, files [hidden, compressed, full, names]
- Hidden – Files information is not obtained.
- Compressed – File contents is shown, max height 200px with scroll
- Full – File contents is shown, no max height, long pages
- Names – Only file names and sizes are shown
GitHub shortcode examples
[github user=f13dev repo=f13-github files=names]
GitHub repository: f13dev/f13-github
Created: April 10, 2021 - 08:27am
Last commit: October 28, 2021 - 06:59pm
Forks: 0
Open issues: 0
Stars: 0
Watchers: 0
Last commit: October 28, 2021 - 06:59pm
Forks: 0
Open issues: 0
Stars: 0
Watchers: 0
Description: GitHub utilities for WordPress
Files (15)
.gitattributes (0.06kb) Download fileREADME.md (0.04kb) Download file
controllers/admin.php (1.64kb) Download file
controllers/control.php (1.45kb) Download file
controllers/gist_shortcode.php (1.07kb) Download file
controllers/git_shortcode.php (1.55kb) Download file
controllers/install.php (0.45kb) Download file
controllers/profile_widget.php (4.55kb) Download file
css/styles.css (3.47kb) Download file
f13-github.php (2.3kb) Download file
models/git_api.php (2.5kb) Download file
views/admin.php (7.64kb) Download file
views/gist.php (4.3kb) Download file
views/profile_widget.php (9.32kb) Download file
views/repo.php (5.35kb) Download file
git clone https://github.com/f13dev/f13-github
No comments on WordPress Plugin: GitHub