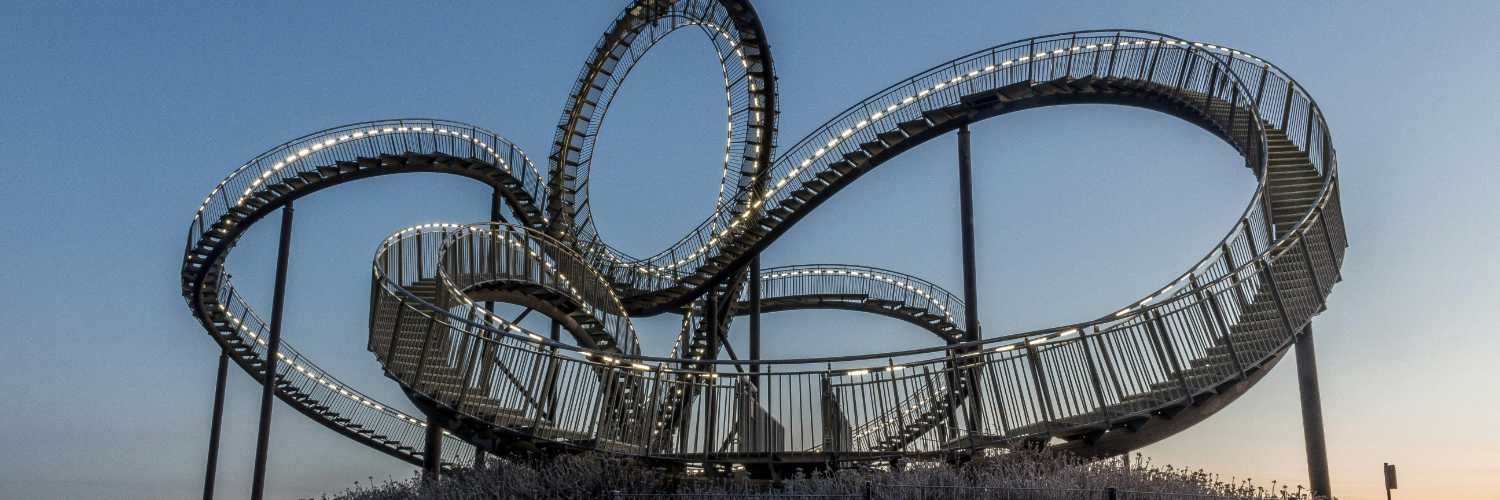
Introduction
Loops are used in programming to iterate over an item, either for a known or unknown amount of times, until a certain criteria has been reached.
You have the choice of writing a single loop and specifying how it ends and writing the same code over and over again to satisfy every iteration of the loop (this will only work if you know the number of times the loop will run). The choice is yours.
<?php $v = 'Count to 10<br>'; $v .= '1<br>'; $v .= '2<br>'; $v .= '3<br>'; $v .= '4<br>'; $v .= '5<br>'; $v .= '6<br>'; $v .= '7<br>'; $v .= '8<br>'; $v .= '9<br>'; $v .= '10<br>'; echo $v;
Or using loops
<?php $v = 'Count to 10<br>'; for ($i = 1; $i <= 10; $i++) { $v .= $i.'<br>'; } echo $v;
Even in this simple example, half the lines of code are required. Imagine if you wanted to count to 100 or even 1,000. The loop would not require any additional lines of code!
While
Run until a condition is true
A while loop will run endlessly until a condition is met, for example the above example in the introduction could also be written as:
<?php $v = 'Count to 10<br>'; $i = 1; while ($i <= 10) { $v .= $i.'<br>'; $i++; } echo $v;
This will achieve the exact same result.
First the “counter” variable needs to be set:
$i = 1;
A while loop is defined, with the condition that $i is less than or equal to 10:
while ($i <= 10) { }
Within the while loop, 2 important things are happening. Firstly the reason for the loop (to count to 10):
$v .= $i.'<br>';
Secondly the “counter” variable is incremented:
$i++;
If the “counter” variable wasn’t incremented, the loop would run endlessly and the condition would never become true.
Do while
Run until a condition is true
Do while offers similar functionality to a While loop with different syntax.
<?php $v = 'Count to 10<br>'; $i = 1; do { $v .= $i.'<br>'; $i++; } while ($i < = 10); echo $v;
For
Run for a pre-determined number of cycles
As you have already seen in the introduction, a for loop looks like:
<?php $v = 'Count to 10<br>'; for ($i = 1; $i <= 10; $i++) { $v .= $i.'<br>'; } echo $v;
There are 2 sections of a for loop to break down, firstly the loop:
for ($i = 1; $i <= 10; $i++) { }
The 3 parameters to a for loop are:
- setting an initial counter variable
- setting a condition to break from the loop
- altering the counter variable on each loop
Secondly the loop contains the desired function for each iteration:
$v .= $i.'<br>';
For each
Run for every item in an array
To explain a foreach loop we will first create an array to iterate over, this array has 4 entries but the loop will work with any number of entries:
<?php $arr = array( array( 'name' => 'John', 'age' => 25, ), array( 'name' => 'Mary', 'age' => 21, ), array( 'name' => 'Peter', 'age' => 19, ), array( 'name' => 'Jane', 'age' => 35, ), );
Iterating over this array and ouputing the name and age of every member in the array can be done like so:
<?php foreach ($arr as $person) { echo $person['name'].': '.$person['age'].'<br>'; }
Will output
John: 25 Mary: 21 Peter: 19 Jane: 35
The loop has 2 important features, firstly defining what is being looped over and setting a temporary variable to access the content for each iteration:
foreach ($arr as $person) { }
For each item in the array variable $arr, create a temporary variable $person. The $person variable is accessible from within the loop. On the first iteration $person will be equal to:
$person = array( 'name' => 'John', 'age' => 25, );
The second feature is the code within the array, continuing the first example for John:
echo $person['name'].': '.$person['age'].'<br>';
will output
John: 25
Continue
Continue will skip the current itteration and continue onto the next. For example, if we wanted to list people from the array above, but only if they were over 22 years old:
<?php foreach ($arr as $person) { if ($person['age'] <= 22) { continue; } echo $person['name'].': '.$person['age'].'<br>'; }
A check is made on the persons age before echoing their name and age. If the age is less than or equal to 22, the loop will continue directly to the next iteration. This example would output:
John: 25 Jane: 35
Break
Break is used to exit the iteration alltogether. Continuing with the person array, we could create a loop that checks if anybody in the array has the name “Peter”:
<?php $peter = false; foreach ($arr as $person) { if ($person['name'] == 'Peter') { $peter = true; break; } } if ($peter) { echo 'Peter is in the array'; } else { echo 'Peter is NOT in the array'; }
The array $arr will be iterated over, if the condition $person[‘name’] == ‘Peter’ is met then the iteration breaks immediately. The above code can be used to determine if anybody in the array is called Peter. Once Peter is found, there is no need to continue with the iteration so we can break from it.
Conclusion
This article covers the basics of iteration in PHP, iteration is similar in many other languages (although there are exceptions). Hopefully this helps to decide which type of loop to use in certain situations.
No comments on PHP Loops