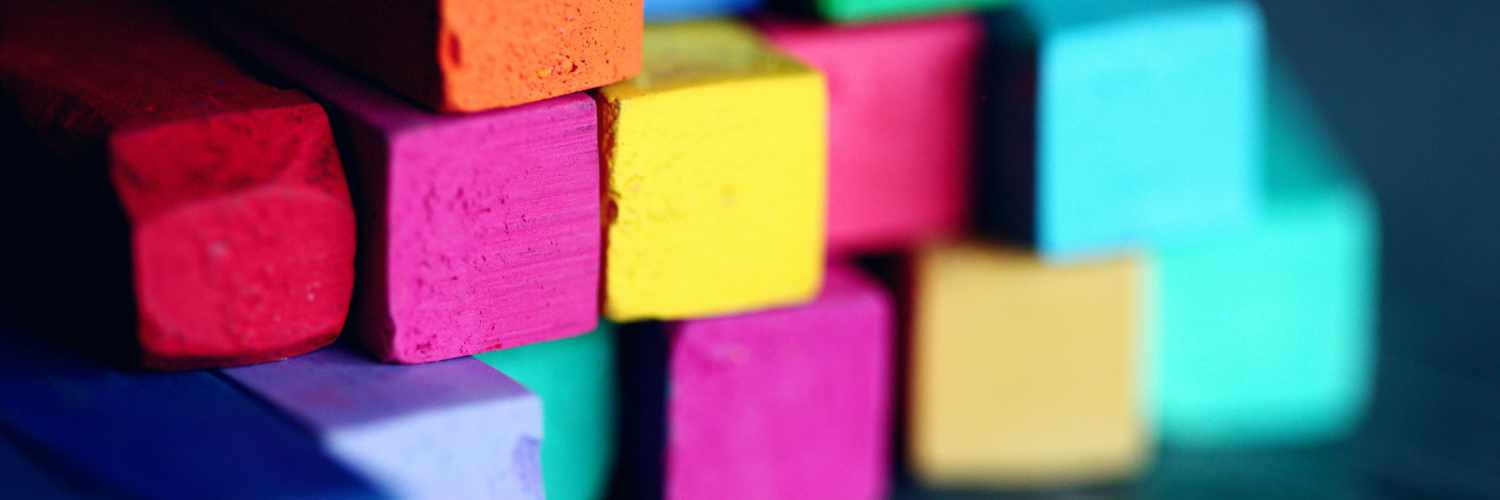
Introduction
Classes and objects are a key principal of OOP (Object-Oriented Programming). The idea behind OOP is that classes are created to represent a real world entity; this real world entity could be a tangible object, such as a person or a car, or an intangible object, such as a job position or a date.
The reason OOP is quite popular is because of the way that it offers code reuse. By writing a class, each instance of the class (or object) uses the same code to operate and store its variables; whereas in procedural programming it is often easy to duplicate much of the code.
Each class is built around a single real world object with methods relating to the real world object. You may have noticed that I am calling these objects, real world object; that is because they are not what is meant by the term ‘object’ in OOP. In OOP and object is what is referred to when an instance of a class has been created.
So, to recap, classes are based on real world objects, whether tangible or intangible; an object is an instance of a class which stores its own data in the variables defined in the class and can utilise the methods or functions as defined in the class.
Each class may have a number of attributes which may primitive data types such as an integer, or of another class; for example an object of the Person class may have an attribute that references an object of a Job class.
Each class will also contain one or more methods, or functions, to create an instance of the class, known as a Constructor; followed by a number of methods, or functions, to retrieve and/or modify each variable.
Examples
Java
/** * A class that represents a person, for each person this class * stores the persons name and their age, in full years */ public class Person { private String name; // An instance variable to store the name of the person private int age; // An instance variable to store the age of the person public Person() { this.name = ""; // Set the name to a default value of an empty string this.age = 0; // Set the age to a default value of 0 } public Person(String aName, int anAge) { this.name = aName; // Set the name to the argument aName this.age = anAge; // Set the age tot he argument anAge } public String getName() { return this.name; // Return the name attribute of the person } public int getAge() { return this.age; // Return the age attribute of the person } public void setAge(int anAge) { this.age = anAge; // Set the age attribute of the person to the argument anAge } public void setName(String aName) { this.name = aName; // Set the name attribute of the person to the argument aName } }
In the above example of a very simple class representing a Person, a number of methods have been created to utilise the attributes of a person object and to update, or set the attributes of a person object. For brevity, JavaDoc has been eliminated.
In this example the class is defined by the following code:
public class Person { // Variables and methods go here }
This tells Java that you are creating a class name Person and all code between the curly brackets ‘{‘ & ‘}’ contain the code that makes up the class. To follow the rules of Java, this class should be saved in a file named ‘Person.java’.
Immediately after the class is defined, a number of instance variables have been declared; each instance variable is initialised for each object of the Person class, and will contain unique values for each object.
private String name; // An instance variable to store the name of the person private int age; // An instance variable to store the age of the person
Next we create a constructor, or in this case, two constructors, known as overloading. The constructors are very similar, but the first one initialises the instance variables for the object to a default value, the second sets the objects instance variables to arguments supplied during construction.
public Person() { this.name = ""; // Set the name to a default value of an empty string this.age = 0; // Set the age to a default value of 0 } public Person(String aName, int anAge) { this.name = aName; // Set the name to the argument aName this.age = anAge; // Set the age tot he argument anAge }
Finally we have created a number of methods, known as setter and getter methods which can be utilised to retrieve, or alter data about the object.
public String getName() { return this.name; // Return the name attribute of the person } public int getAge() { return this.age; // Return the age attribute of the person } public void setAge(int anAge) { this.age = anAge; // Set the age attribute of the person to the argument anAge } public void setName(String aName) { this.name = aName; // Set the name attribute of the person to the argument aName }
You may have noticed that Java methods have two key words before the method name; the first of which is an access modifier, public access allows the method to be called from anywhere in the system, private access will only allow the method to be called from within the same class, or the default modifier (which is set by not providing an access modifier) will allow the method to be called from within any class in the same package.
The second key word, as seen above as ‘String’, ‘int’ or ‘void’ specifies the return type of the method; the first method ‘public String getName()’ returns a value that is a String. The second method ‘public int getAge()’ returns an integer and the final two methods do not return a value, so they use the return type ‘void’.
In order to utilise this class we can:
// Create a new person object, named person1, using the default age and name values Person person1 = new Person(); // Create a new person object, named person2, setting the name and age values using arguments Person person2 = new Person("John Doe", 42); // Update the name of person1 person1.setName("Jane Doe"); // Update the age of person1 person1.setAge(44); // Retrieve data about person1 System.out.println("Person1 is called: " + person1.getName()); // This will print "Person1 is called: Jane Doe" to the shell // Retrieve data about person2 System.out.println("Person2 is " + person2.getAge() + " years old"); // This will print "Person2 is 42 years old" to the shell
We could now add a new method to the Person class to account for a person object having a birthday. If we were to add the following code, before the final closing curly bracket ‘}’:
public void hasBirthday() { this.setAge(this.getAge() + 1); }
What this method effectively does, is set the persons age to their current age + 1.
Now continuing from the above example:
// Create a new person object using arguments to set the persons name and age Person person2 = new Person("John Doe", 42); // person2 celebrates a birthday person2.hasBirthday(); // Print information about person2 System.out.println("Person2 is " + person2.getAge() + " years old"); // This will print "person2 is 43 years old" to the shell
PHP
<?php class Person { $name = ''; // Variable to store the name of the person $age = 0; // Variable to store the age of the person function __construct($aName, $anAge) { // A constructor to set the persons name and age to the values of the arguments $this->name = $aName; // Set the name of the person to the value of the argument $aName $this->age = $anAge; // Set the age of the person to the value of the argument $anAge } function getAge() { return $this->age; // Return the age of the person } function getName() { return $this->name; // Return the name of the person } function setAge($anAge) { $this->age = $anAge; // Set the persons age to the value of the argument $anAge } function setName($aName) { $this->name = $aName; // Set the persons name to the value of the argument $aName } function hasBirthday() { $this->age = $this->getAge() + 1; // Increment the persons age by 1 } }
The above code represents the Java example class, but represented in PHP to show the differences in syntax. A number of differences can be noted:
- PHP does not support overloaded functions, therefor only one constructor has been implemented
- The constructor is defined with ‘__construct()’ rather than the Java equivalent of ‘public Person()’
- PHP does not require a return type to be specified, this is because PHP classes all variables as the same, they are not limited to a type.
- Each method, or function starts with he keyword ‘function’.
- PHP does not require an access modifier, instead, not setting an access modifier results in the PHP equivalent of public access, alternatively a private function can be created using the keyword ‘private’ before ‘function’.
- Java references instance variables with ‘this.variableName’, PHP references variables with ‘$this->variableName’
Using the above code objects can be created and manipulated like so:
<?php // Create two person objects, setting the name and age via arguments $person1 = new Person("", 0); $person2 = new Person("John Doe", 42); // Update the name of person1 $person1->setName("Jane Doe"); // Update the age of person1 $person1->setAge(44); // Retrieve data about person1 echo 'Person1 is called: ' . $person1->getName(); // This will echo 'Person1 is called Jane Doe' // Retrieve data about person2 echo 'Person2 is ' . $person2.getAge() . ' years old'; // This will echo 'Person2 is 42 years old' // Person 2 has a birthday, their age is incremented by 1 $peson2->hasBirthday(); // Retrieve data about person2 echo 'Person2 is ' . $person2.getAge() . ' years old'; // This will echo 'Person 2 is 43 years old'
Conclusion
Hopefully from this article you will have gained, or improved your current knowledge on the following:
- Understand what a class and an object are
- Understand what methods, functions and constructors are
- Have a basic knowledge of access modifiers
- Have a basic knowledge of Java return types
- Understand how a class is constructed and how objects are created from the class
No comments on OOP: Writing a class and creating objects