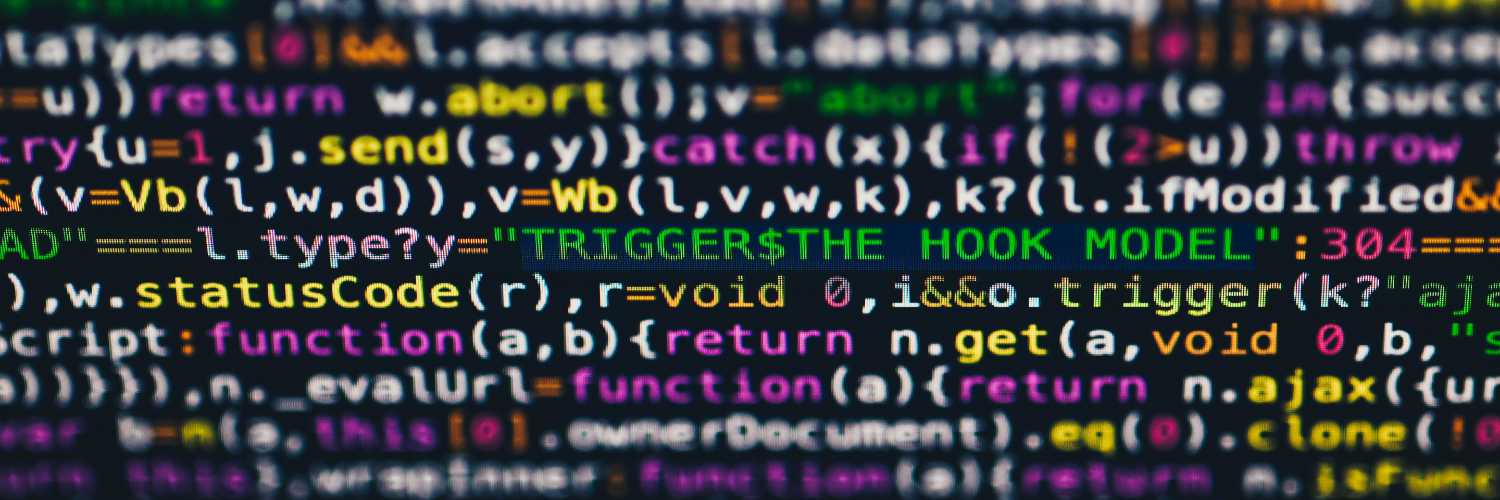
Introduction
Traditional web applications reload the whole page when clicking a link, modern web applications only reload the content that has changed.
As the web has matured, web applications often have a large amount of overhead; reloading every aspect of the page on each click including JavaScript and CSS vastly increases the overheads.
Using AJAX to update content as it changes rather than the whole page helps reduce the modern web overhead.
Switching content with AJAX
Although maybe not the most exciting part of AJAX, simple switching of static content is a good place to start. In this section you will see how links can be over ridden by jQuery to load static content into a section of the page in the most basic form.
HTML
For this example, we will have 3 HTML files, index.html, content1.html and content2.html.
index.html
<!DOCTYPE html> <head> <script src="jquery.min.js></script> <script src="ajax.js"></script> </head> <body> <a class="ajax-link" data-href="content1.html" data-target="page-container">Content 1</a> <a class="ajax-link" data-href="content2.html" data-target="page-container">Content 2</a> <div id="page-container"></div> </body>
content1.html
<p> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Maecenas volutpat dignissim efficitur. Donec vitae est sit amet quam suscipit pretium. Sed justo erat, vehicula et. </p>
content2.html
<p> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vivamus suscipit et nulla in tristique. Maecenas ullamcorper, dui ut scelerisque laoreet, augue mauris posuere nunc, ut. </p>
jQuery
ajax.js
jQuery(document).ready(function($) { $(document).on('click', '.ajax-link', function(e) { e.preventDefault(); var target = '#'+$(this).data('target'); var url = $(this).data('href'); $.ajax({ type : 'GET', url : url, }).done(function(content) { $(target).html(content); }).error(function(error) { alert('An error occured.'); }); }); });
Breakdown
When the document is ready
jQuery(document).ready(function($) { // functions });
When a user clicks on an element with the class “ajax-link”
$(document).on('click', '.ajax-link', function(e) { // Do something });
Stop the intial function of the event, for example, this will stop the href attribute of an anchor tag being loaded.
e.preventDefault();
Setting variables, this doesn’t necessarily have to be done, it just makes things simpler
var target = '#'+$(this).data('target'); var url = $(this).data('href');
Do the AJAX, “type” is set to GET, POST can also be used.
“url” is set to the variable “url”, in this case either content1.html or content2.html
If the AJAX request was successful, the “done” function will run, otherwise the “error” function will run. In this case the done function takes the loaded content and inserts it into the target div, the error function provides an alert message.
$.ajax({ type : 'GET', url : url, }).done(function(content) { $(target).html(content); }).error(function(error) { alert('An error occured.'); });
Conclusion
From following this short guide, you have learnt how to use AJAX to switch certain content in the page without reloading the whole page.
This is of course a very basic guide. In the next chapters we will discuss loading dynamic content, processing form POST data and integration with WordPress.
No comments on AJAX basics – Switching static content