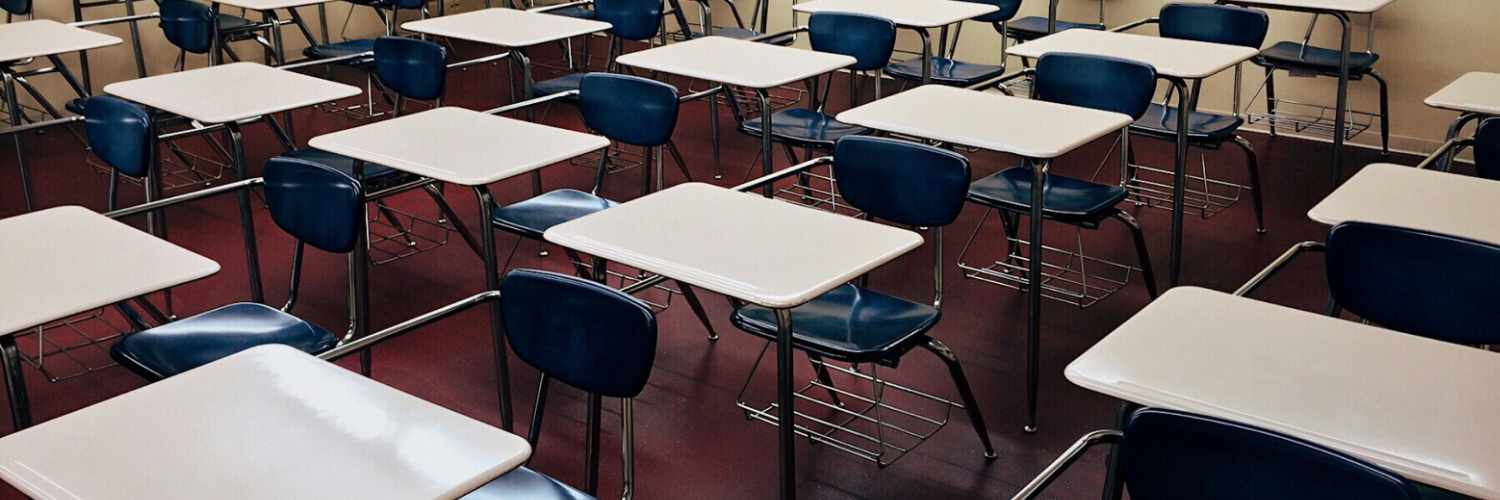
Introduction
Initialising classes in Object-Oriented Programming is something that will be done often, I guess it’s part and parcel of OOP and the whole point of it.
Have you ever considered that there are more efficient ways to initialise a class and not all are equal. Depending on how you want to use the class or how often it is used, improvements can be made to reduce memory usage.
Init on demand
When referencing classes, the class is moved into working memory as an object. If a class requires 2MB of memory to be loaded and it is loaded 10 times, 20MB of memory is used.
For our starting point, we will create an example class that has 2 functions and loads a Helper class twice, once for each function.
<?php
class Example
{
public function __construct()
{
//
}
public function stepOne()
{
$helper = new Helper();
$helper->processStepOne();
$this->stepTwo();
}
public function stepTwo()
{
$helper = new Helper();
$helper->processStepTwo();
}
}
Although this is our starting point that we are going to improve on, there are instances where we would want to initialise a class multiple times. If we are initialising a class with different parameters, there is little we can do to improve this.
Init once, use many
We can easily alter the above Example class so that the Helper class is only loaded once by initialising it in the constructor.
Now we have saved the processing and memory resources by only loading this class once.
<?php
class Example
{
private $helper;
public function __construct()
{
$this->helper = new Helper();
}
public function stepOne()
{
$this->helper->processStepOne();
$this->stepTwo();
}
public function stepTwo()
{
$this->helper->processStepTwo();
}
}
Init once, use many, on demand
Now that our Example class has been fixed to only load the Helper class once for both functions, let’s throw a spanner into the works.
If we add a third function to the Example class that does not reference the Helper class, we will still be loading the Helper class into memory even if we never use it. Now we’re wasting memory and processing where it’s not required. To fix this, we can create an Init once, use many constructor.
Here we introduce the init once, use many, on demand method.
<?php
class Example
{
private $helper
public function __construct()
{
//
}
public function stepOne()
{
$this->helper()->processStepOne();
$this->stepTwo();
}
public function stepTwo()
{
$this->helper()->processStepTwo();
}
private function helper()
{
if (!($this->helper instanceof Helper)) {
$this->helper = new Helper();
}
return $this->helper;
}
}
Here the new helper() function is called instead of directly calling the variable that stores the Helper class.
If the helper variable isn’t an instance of the Helper class, it is initialised then returned, if the variable is already set, it is returned without initialising a new copy of the Helper class.
Conclusion
In this article we have discussed alternative methods of loading classes depending on how we intend to use the class:
- Classes are initialised with bespoke parameters – Init on demand
- All functions use the helper class – Init once, use many
- More than one function uses the helper class, but not all – Init once, use many, on demand
Using these methods, PHP transaction memory usage can be reduced as only a single copy of each class is loaded into memory, unless multiple instances are required.
No comments on Optimising PHP: Class initialisation