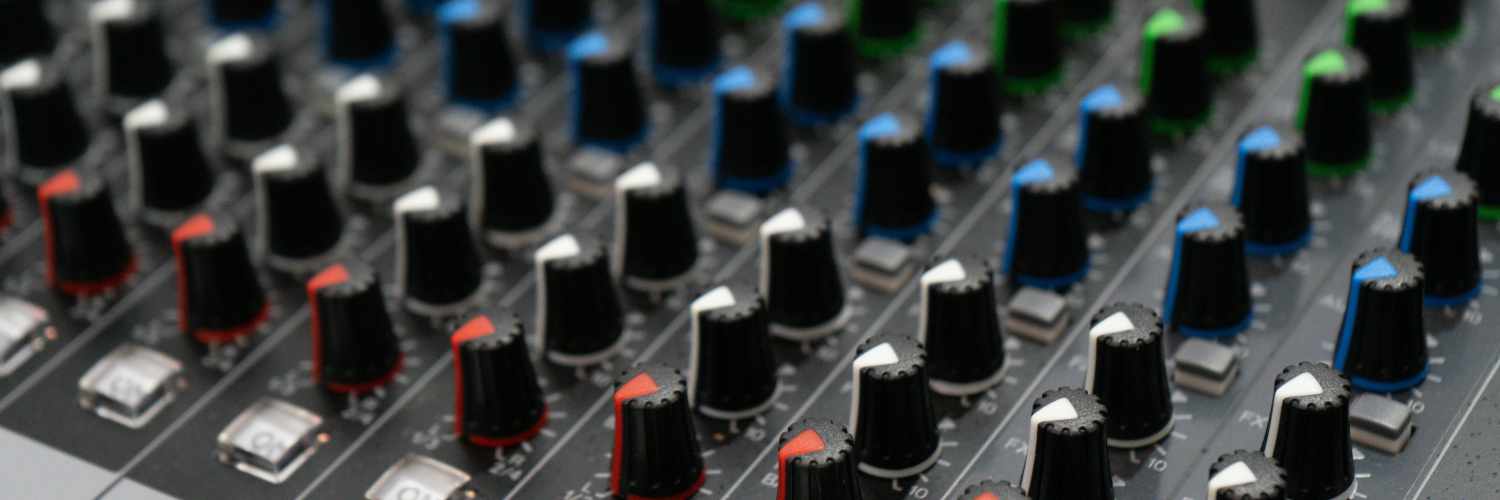
Introduction
Variables form an essential part of every application for storing, retrieving and generating dynamic data. In OOP (Object-Oriented Programming) there are three main types of variables which provide slightly different functionality; Instance variables, Class variables and Local variables.
Instance variables
An instance variable is a variable associated with an instance of a class, known as an object. Each instance of the same class will have the same set of variables, although with their own unique values.
Using a Person class for an example, each object of the Person class will have an instance variable for name and age, each objects name and age attributes will be unique to the object.
Class variables
A class variable is a variable associated with the class as a whole, it is not assigned as an attribute of each instance of the class and only one value is stored in the class variable, no matter how many instances of the class exist.
Again, using a Person class as an example, a class variable could be introduced to record the population; each time a Person object is created, a line of code in the constructor would increment the class variable for population; similarly, each time an object of the Person class records a death the population class variable would be decremented.
Local variables
A local variable is a variable that is only accessible within a certain method or constructor. Local variables are used by methods to store any actual arguments that are sent as part of the message received by the method.
Back to the example of a Person class, when the message setAge(52) is sent to an object of the Person class, the integer value ’52’ will be stored in a local variable. This local variable is only accessible by the method from which it was created, and is destroyed once the method has completed its duty.
Example
Code (Java)
/** * A class that represents a person, for each person this class * stores the persons name and their age, in full years */ class Person { private static int population = 0; // A class variable to record the population private String name; // An instance variable to store the name of the person private int age; // An instance variable to store the age of the person public Person() { this.name = ""; // Set the name to a default value of an empty string this.age = 0; // Set the age to a default value of 0 // Increment the population Person.population = Person.population + 1; } public Person(String aName, int anAge) { this.name = aName; // Set the name to the argument aName this.age = anAge; // Set the age tot he argument anAge } public String getName() { return this.name; // Return the name attribute of the person } public int getAge() { return this.age; // Return the age attribute of the person } public void setAge(int anAge) { this.age = anAge; // Set the age attribute of the person to the argument anAge } public void setName(String aName) { this.name = aName; // Set the name attribute of the person to the argument aName } public void registerDeath() { // Change the persons name and age to represent a deceased person this.name = "Deceased"; this.age = -1; // Decrement the population by 1 Person.population = Person.population - 1; } @Override public String toString() { String aString = "A person named: " + this.getName() + " who is " + this.getAge() + " years old"; return aString; } }
Identifying variables
Although the above example doesn’t actually remove an object when it receives the message ‘registerDeath()’ this class is suitable to explain the differences in variable types. In order to actually remove the deceased object we would require a map, set or list, which is beyond the scope of this tutorial.
The current state of the Person class as above includes a number of variables; at least one of each type.
Instance variables are declared in the opening of the Person class and include:
- private String name
- private int age
A class variable is declared in the opening of the Person class and is defined by the preceding keyword ‘static’. The class variable is:
- private static int population
A number of local variables have been utilised in the Person class, most of which are declared in the method signature, such as the following from the Person constructor:
- String aName
- int anAge
Another local variable has been declared in the newly added toString() method; where the String to be returned is first stored in a local variable before being returned.
- String aString = “…”
Declaration and access
As can be seen from the above code example, declaration and access varies depending on the type of variable being used. Both instance variables and class variables are declared at the top of the main body of the class, outside of any methods or constructors; both instance and class variables are usually declared as private to aid in data hiding. A class variable is proceeded by the keyword ‘static’ which tells Java that the variable is static across all objects of the class.
Local variables can either be declared in the method signature as an argument, or individually in the body of the method. In the method signature ‘setName(String aName)’, a String variable is declared called aName; this variable is only accessible from within the method it is declared. Another way to use a local variable is to declare it in a similar way to that of an instance variable, but within the body of a method. A local variable does not require an access modifier such as ‘private’ as it is only accessible within the method it is initialised.
In order to access an instance variable, only the name of the variable is required; although it is good practice to proceed the name with the keyword ‘this’ followed by a full stop. Using the keyword ‘this’ makes it clear that the variable being accessed is a part of the same object. Similarly an instance variable can be accessed using the object name followed by a full stop, for example to access the name of a Person object named ‘person1’ the variable could be accessed with ‘person1.name’, or a Person object may access it’s own name variable with ‘this.name’; although in this example an object can only access its own name variable due to the private access modifier of the name variable.
A class variable is not specifically a part of an object and thus cannot be accessed using the keyword ‘this’; instead, as it is part of the class it is accessed by preceding the variable name with the class name and a full stop. In the Person class, the population class variable can be accessed via ‘Person.population’.
To access a local variable, only the name of the variable is required; using the keyword ‘this’, would imply that the variable is a part of the object, whereas it is not; it is simply a part of a method in the class.
Conclusion
From this post you should hopefully have gained an understanding of the different types of variables available in Object-Oriented Programming and the associated keyword used by Java.
No comments on OOP: Instance variables, Class variables & Local variables