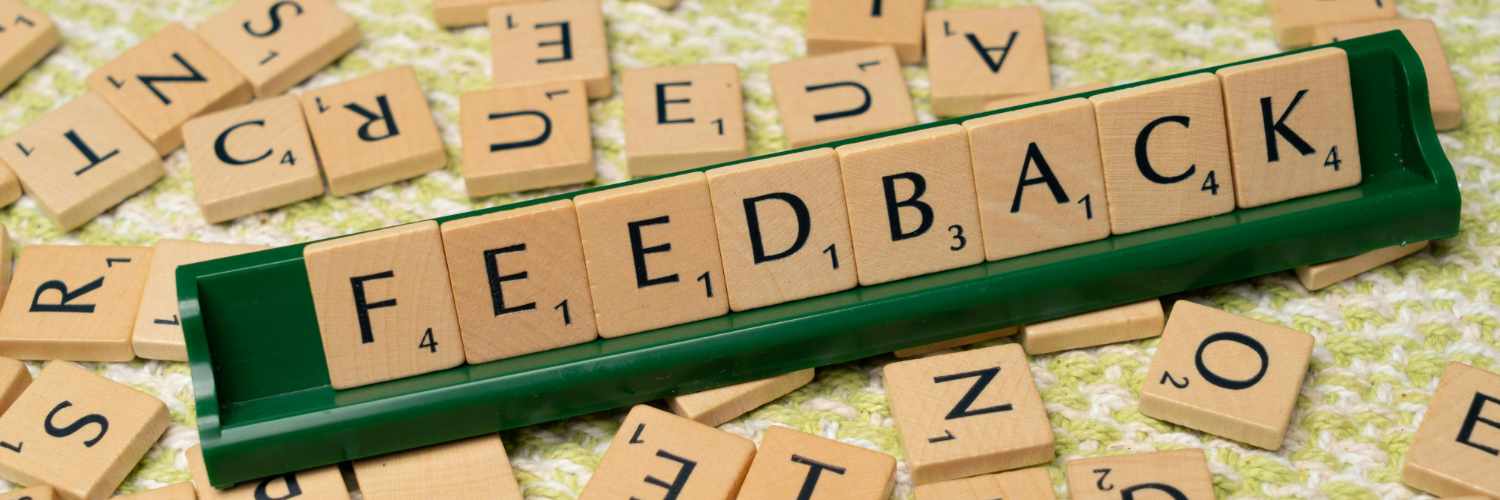
Introduction
What is a code comment?
Code comments offer a method of annotation for the code that makes up an application, a part of an application, or simply a single line of code. When source code is compiled, or executed comments are ignored; they have no affect on the final product, the output of a method, or what a line of code produces.
Why are code comments useful?
With anything where the basic syntax may not be immediately readable to others, or even yourself when looking back; annotation offers simplistic plain English (or any other language) explanations of what the intention of the code is. Even when seasoned programmers look back on code that they have written days, months, or years ago, the intention may still not be immediately obvious. For this reason programmers add annotation, or comments between code to explain the intention of the code, how it works, or even what is wrong with it; this annotation helps the programmer to understand their own intentions.
Comments are especially important when writing open source applications; others who read or contribute to the code may not be as experienced, or may be used to a different coding style. In this situation annotation helps another person better understand what the code does.
Examples
Virtually every programming and scripting language offers a method of annotation; although the methods sometimes differ. Below are a series of examples of how comments are added.
HTML & CSS
<!DOCTYPE html> <html> <head> <title>My page title</title> <style type="text/css"> h1 { /* Change the colour to grey */ color: #DDD; <!-- Change the line height to 1.5em --> line-height: 1.5em; } </style> </head> <body> <!-- Put the page title here --> <h1>Page title</h1> <!-- Put the page content underneath --> </body> </html>
In the example above, although very simple and maybe a little overkill adding annotation, a number of comments have been included which will not affect the page that is displayed by a web browser.
Within HTML code comments can be added using an opening tag of ‘<!–‘ and a closing tag of ‘–>’, the comment can be on the same line as code and anything between the opening and closing tag are not included in the visual output; although anybody who selects to view the page source will be able to see the comments.
CSS can utilise the same commenting tags as HTML, but can also utilise another type of commenting tag. Similar to the HTML comment tags, anything between the opening tag of ‘/*’ and the closing tag ‘*/’ will not affect the output; but again will be visible by viewing the page source.
Java
/* a class that represents a person */ public class Person { private int age; // The age of the person private String name; // The name of the person /** * Constructor for a person, setting the age and name of the person * to the values of the arguments. * @Param anAge The age of the person * @Param aName The name of the person */ public Person(int anAge, String aName) { this.age = anAge; // Set the age attribute to the argument anAge this.name = aName; // Set the name attribute to the argument aName } }
In the above example, an incomplete class modelled on a person shows three different types of comments. The first shows the same commenting syntax as that of CSS, where anything between the opening tag of ‘/*’ and ‘*/’ is omitted when the application is compiled. The second method of commenting is opened with the ‘//’ tag, this method of comment is slightly different as it doesn’t explicitly have a closing tag, in reality the closing tag is the end of the line; once a new line is started the comment is ended.
The third method of annotation in Java is similar to the first, although this time opening with the tag ‘/**’ and each further line starting with a single ‘*’, in Java this form of annotation is known as JavaDoc, comments included within this syntax are used by Java to automatically generate documentation for the class.
PHP
<?php # An instance variable to store the persons age $age = 42; // An instance variable to store the persons name $name = 'John Doe'; /* Print the persons name and age. */ print($name . ' ' . $age);
PHP allows for three methods of annotation, two of which are single line; as in they are ended by starting a new line. A single line PHP comment can be started with either the c++ opening tag ‘//’, or the shell script opening tag ‘#’; any text or code after the opening tag, but on the same line, will be ignored when the code is executed.
Similar to Java and CSS, as above, PHP can also utilise the multi-line comment tags, opened with’/*’ and closed with ‘*/’; any text or code between these tags, even spanning multiple lines, will not be executed.
As PHP is generally used for creating dynamic web applications it is probably worth mentioning that PHP comments will not be displayed when viewing the web pages source code via a web browser.
Conclusion
From this article you should have acquired an understanding of why comments are written within an applications source code and some of the methods used to write comments in programming languages.
If you are working with a different language than those listed above, it should now be fairly simple to find instruction of the commenting syntax used; as hundreds of programming languages exist, it would be impractical to list an example for each.
Should you require any help with further understanding code comments, then please leave a message below.
No comments on Commenting code